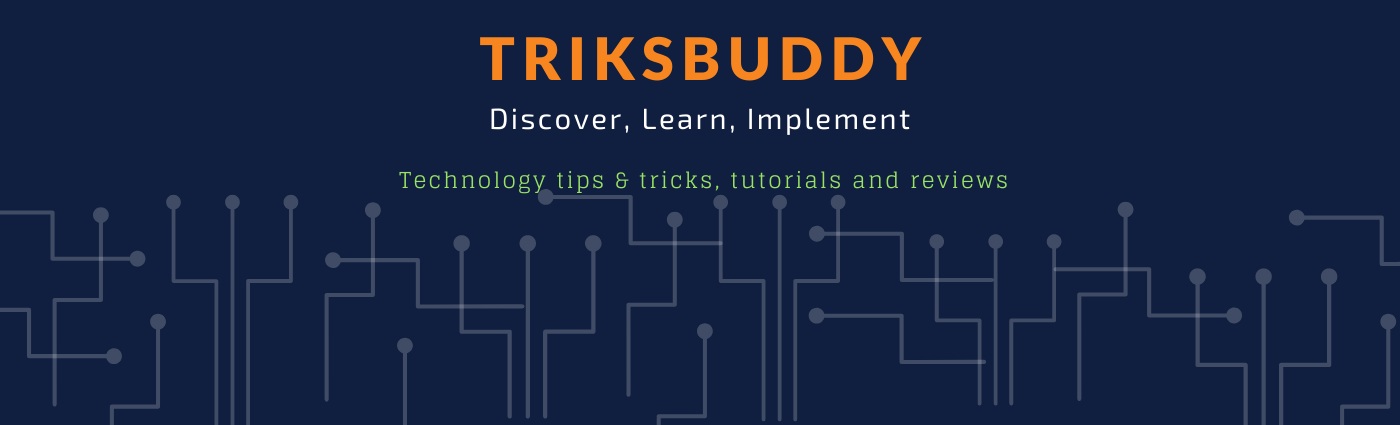
Welcome to triksbuddy blog. He we discuss on different technology, tips and tricks, programming, project management and leadership. Here we share technology tutorials, reviews, comparison, listing and many more. We also share interview questions along with answers on different topics and technologies. Stay tuned and connected with us and know new technologies and dig down known ones.
Friday, March 24, 2023
How to implement async CRUD methods for an Employee model using Dapper with Oracle
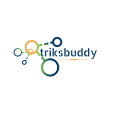
How to implement async CRUD methods for an Employee model using Dapper with MySQL
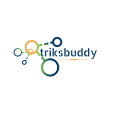
How to implement async CRUD methods for an Employee model using Dapper with PostgreSQL
You will need to install and add reference to the Npgsql package to communicate with PostgreSQL database.
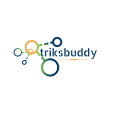
Thursday, March 23, 2023
How to read data in .net from Oracle using Dapper
Note that you will need to add the reference to the Oracle.ManagedDataAccess.Client package.
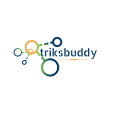
Different ways to write a method that reads list of entities from SQL Server in C# language
There are different ways we can do same work. With evolution of .net technologies we have found different technologies to perform data operations. Here we will look at different ways of getting a list of employees from SQL Server database using different data access technologies.
Method-1: using ADO.NET
This method retrieves the employee list from the "Employee" table in the SQL Server database using a SELECT query, and maps the data to a list of Employee objects. Note that in this example, the Employee class has properties for the Id, Name, Department, and Salary fields in the database, and the SqlDataReader's Get methods are used to retrieve the values for each field from each record.
Method-2: using ADO.NET async
In this async version of the method, the SqlConnection is opened asynchronously using await connection.OpenAsync(), and the SqlDataReader is created and executed asynchronously using await command.ExecuteReaderAsync(). The while loop that reads the data is also made asynchronous using await reader.ReadAsync(). Note that the using statements are still used to ensure proper disposal of resources.
Method-3: using Dapper
In this async version of the method, the SqlConnection is opened asynchronously using await connection.OpenAsync(). The query is then executed asynchronously using Dapper's QueryAsync method, which returns a list of Employee objects already mapped to the data returned by the SQL query. The using statement is still used to ensure proper disposal of resources.
In this async version of the method, a DbContextOptionsBuilder is used to configure the connection string and then used to create a new DbContext instance. The Set method is used to retrieve the DbSet<Employee>, and then the ToListAsync method is called to execute the query asynchronously and retrieve the Employee objects already mapped to the data returned by the SQL query. The using statement is still used to ensure proper disposal of resources. Note that you will need to add the appropriate NuGet packages and configure EF Core for your specific database provider.
Method-5: LINQ to SQL:
In this async version of the method, a DataContext is used to connect to the database and a Table<Employee> is used to retrieve the table of Employee objects. The ToListAsync method is called on the Table<Employee> to execute the query asynchronously and retrieve the Employee objects already mapped to the data returned by the SQL query. Note that you will need to add the appropriate references to System.Data.Linq and configure the DataContext for your specific database provider. The using statement is still used to ensure proper disposal of resources.
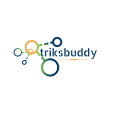