- Use asynchronous programming: Asynchronous programming can improve the scalability of your Web API by allowing it to handle more concurrent requests. Use async/await and Task-based programming to make sure your Web API is responsive and efficient.
- Optimize database queries: Optimizing database queries can help to improve the performance of your Web API by reducing the number of queries that need to be executed.
- Use database connection pooling: Database connection pooling can help improve the performance of your Web API by reducing the time it takes to establish a connection to the database. By reusing existing connections, you can avoid the overhead of establishing new connections, which can significantly improve performance.
- Use efficient data structures and algorithms: Using efficient data structures and algorithms can help improve the performance of your Web API. By choosing the right data structures and algorithms, you can reduce the time it takes to perform operations and improve the overall performance of your Web API.
- Implement pagination: When returning large data sets, it's important to implement pagination to improve the performance of your Web API. Use query parameters to allow clients to specify the page size and page number, and use the Skip and Take methods in LINQ to retrieve the correct data.
- Use DTOs (Data Transfer Objects): DTOs are objects that carry data between different layers of your application, such as between your Web API and your database. Use DTOs to avoid exposing your domain objects to the outside world, and to provide a clear contract between your Web API and its clients.
- Use unit tests and integration tests: Unit tests and integration tests can help you identify issues in your Web API early on, before they become bigger problems. Use a testing framework that suits your application's needs, such as xUnit or NUnit.
- Implement caching: Caching can greatly improve the performance of your Web API by reducing the number of requests to your database or other data sources. Use a caching strategy that suits your application's needs, such as in-memory caching, distributed caching, or client-side caching.
- Use a distributed cache: A distributed cache can help improve the scalability of your Web API by distributing the caching across multiple servers. By using a distributed cache, you can avoid overloading any one server and ensure that your Web API can handle a large number of requests.
- Use HTTP compression: HTTP compression can help to reduce the size of the data being transferred, which can help to improve the performance of your Web API.
- Use a content delivery network (CDN): A CDN can help to improve the scalability of your Web API by caching content and delivering it from the closest edge server to the user.
- Use containerization: Containerization can help to improve the scalability of your Web API by allowing you to quickly and easily spin up new instances of your application as demand increases.
- Use a distributed architecture: A distributed architecture can
help to improve scalability by allowing you to distribute the load
across multiple servers or nodes.
- Use a load balancer: A load balancer can distribute incoming requests across multiple servers, improving the scalability and availability of your Web API. Use a load balancer that suits your application's needs, such as a hardware load balancer or a software load balancer like NGINX.
- Implement rate limiting: Rate limiting can prevent clients from making too many requests to your Web API, which can help prevent denial-of-service attacks and improve the overall performance of your Web API. Use a rate limiting strategy that suits your application's needs, such as token bucket or fixed window rate limiting.
- Use HTTPS: HTTPS encrypts the data transmitted between your Web API and its clients, improving the security and privacy of your application. Use a trusted SSL/TLS certificate and configure your Web API to use HTTPS.
- Use an API gateway: An API gateway can provide a single entry point for your Web API, allowing you to manage and secure your API more easily. Use an API gateway that suits your application's needs, such as AWS API Gateway or Azure API Management.
- Use a message queue: A message queue can help to improve the scalability of your Web API by allowing you to process requests asynchronously.
- Use performance monitoring: Performance monitoring can help you identify performance issues in your Web API and improve its scalability. Use a performance monitoring tool that suits your application's needs, such as Application Insights or New Relic.
- Keep it simple: Finally, it is important to keep your Web API simple and easy to understand. Use clear and concise code, follow best practices, and keep the API focused on its core functionality.
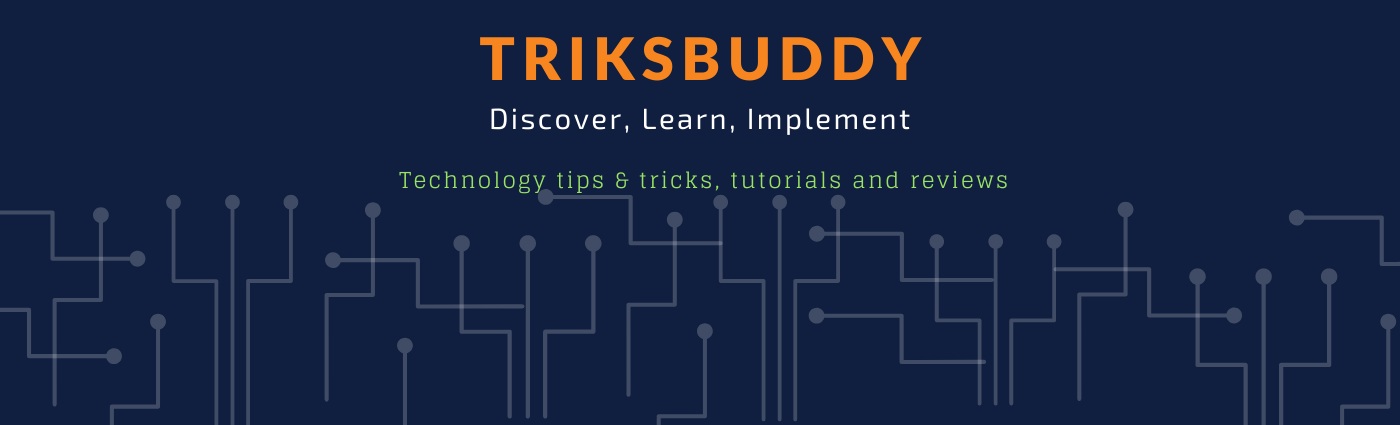
Welcome to triksbuddy blog. He we discuss on different technology, tips and tricks, programming, project management and leadership. Here we share technology tutorials, reviews, comparison, listing and many more. We also share interview questions along with answers on different topics and technologies. Stay tuned and connected with us and know new technologies and dig down known ones.
Wednesday, April 19, 2023
What are some best practices for designing and building a scalable .NET Core Web API?
Here are some best practices for designing and building a scalable .NET Core Web API:
Labels:
.NET Core,
Interview Question,
Web API
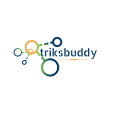
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment
Please keep your comments relevant.
Comments with external links and adult words will be filtered.