Cross-Origin Resource Sharing (CORS) is a mechanism that allows web applications running in one domain to access resources in another domain. CORS is enforced by web browsers to protect users from malicious attacks, such as cross-site scripting (XSS) and cross-site request forgery (CSRF).
In ASP.NET Core, you can enable CORS by adding the Microsoft.AspNetCore.Cors package to your project and configuring it in the Startup.cs file.
Here's an example of how to enable CORS for all requests in your application:
1. Install the Microsoft.AspNetCore.Cors package:
dotnet add package Microsoft.AspNetCore.Cors
2. Add the CORS middleware to the application pipeline:
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseCors(builder =>
builder.AllowAnyOrigin()
.AllowAnyHeader()
.AllowAnyMethod());
// ...
}
This code enables CORS for all requests by allowing any origin, any header, and any method.
You can also specify more specific CORS settings by using the CorsPolicyBuilder class:
public void ConfigureServices(IServiceCollection services)
{
services.AddCors(options =>
{
options.AddPolicy("AllowSpecificOrigin",
builder =>
{
builder.WithOrigins("http://example.com")
.AllowAnyHeader()
.AllowAnyMethod();
});
});
// ...
}
This code enables CORS for requests from http://example.com by allowing any header and any method.
By default, ASP.NET Core uses the AllowAnyOrigin method to allow requests from any domain. However, you can configure CORS to allow requests only from specific domains or with specific headers and methods.
Enabling CORS in your ASP.NET Core application can be useful when your client-side JavaScript code needs to make requests to a server in a different domain or port. However, you should always be careful when enabling CORS and ensure that your server-side code is properly secured against malicious attacks.
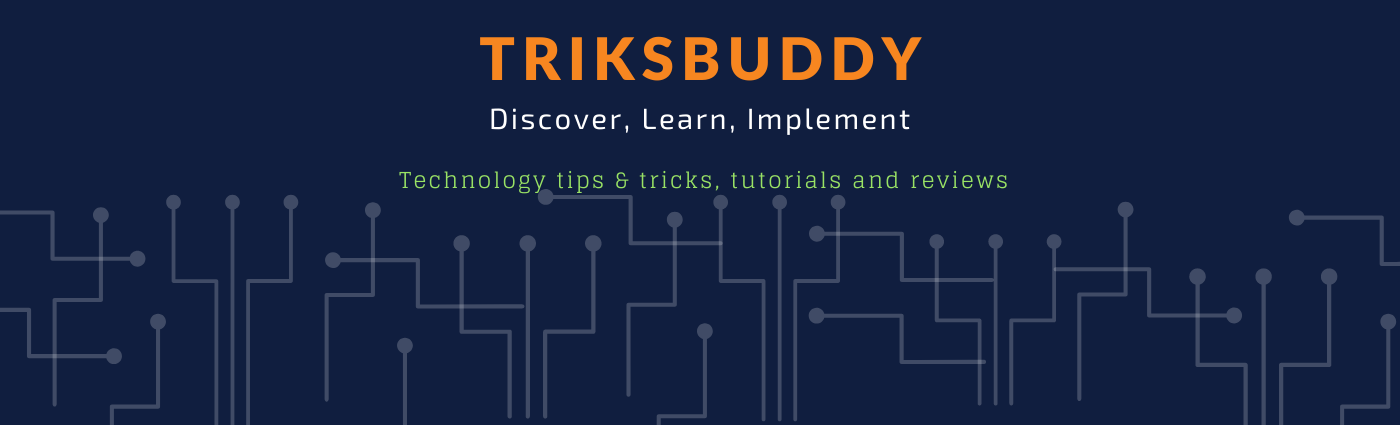
Welcome to triksbuddy blog. He we discuss on different technology, tips and tricks, programming, project management and leadership. Here we share technology tutorials, reviews, comparison, listing and many more. We also share interview questions along with answers on different topics and technologies. Stay tuned and connected with us and know new technologies and dig down known ones.
Saturday, April 15, 2023
What is CORS and how do you enable it in ASP.NET Core?
Labels:
.Net,
.NET Core,
Interview Question
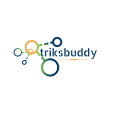
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment
Please keep your comments relevant.
Comments with external links and adult words will be filtered.