In unit testing, a test fixture is a set of objects and resources that are used to set up and execute a test. It provides a known state and environment for the test to run in, and ensures that the test is run consistently and reliably.
In .NET Core, test fixtures are typically implemented using a test class with a set of test methods. Each test method represents a specific scenario or test case, and is designed to test a specific feature or behavior of the system being tested.
Here's an example of how to use a test fixture in unit testing:
Suppose you have a class Calculator with a method Add that performs addition of two integers. To test the Add method, you can create a test fixture that sets up the environment and executes the test:
public class CalculatorTests
{
private Calculator _calculator;
[SetUp]
public void Setup()
{
// Create a new instance of the Calculator class
_calculator = new Calculator();
}
[Test]
public void Add_WhenCalled_ReturnsSumOfArguments()
{
// Arrange
int x = 2;
int y = 3;
// Act
int result = _calculator.Add(x, y);
// Assert
Assert.AreEqual(5, result);
}
}
In this example, the CalculatorTests class is a test fixture that contains a set of test methods for the Calculator class. The Setup method is decorated with the [SetUp] attribute, which indicates that it should be run before each test method. The Setup method creates a new instance of the Calculator class, which ensures that the test is run in a clean environment.
The Add_WhenCalled_ReturnsSumOfArguments method is a test method that represents a specific scenario or test case for the Add method. The Arrange section sets up the initial state and inputs for the test, the Act section performs the operation being tested, and the Assert section verifies that the result of the operation is correct.
By using a test fixture, you can ensure that each test is run in a known environment and that the results are consistent and reliable. This can help you to identify and fix issues early in the development process, and ensure that your code is of high quality and meets the requirements and specifications.
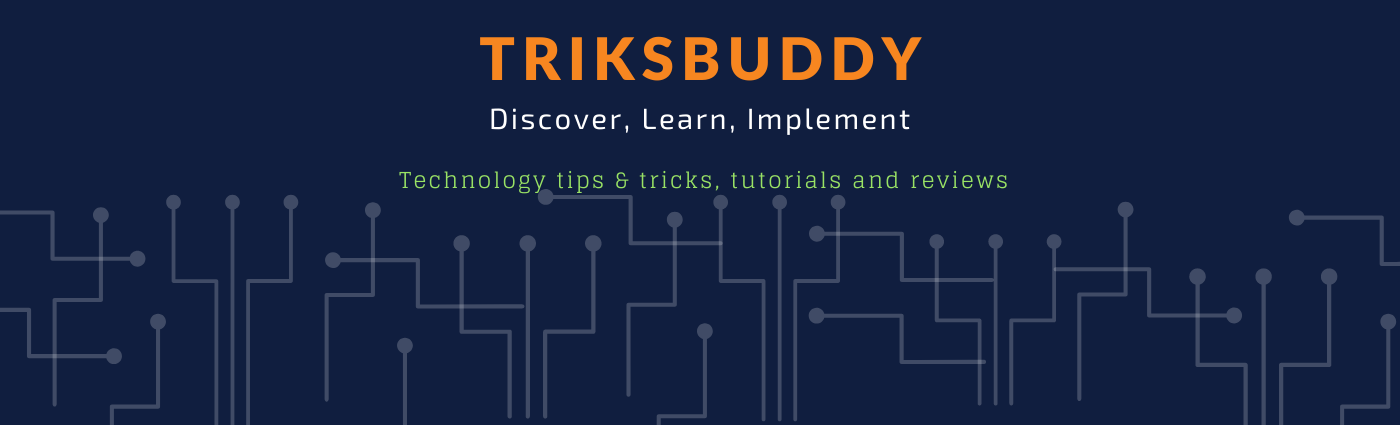
Welcome to triksbuddy blog. He we discuss on different technology, tips and tricks, programming, project management and leadership. Here we share technology tutorials, reviews, comparison, listing and many more. We also share interview questions along with answers on different topics and technologies. Stay tuned and connected with us and know new technologies and dig down known ones.
Monday, April 17, 2023
What is a test fixture and how do you use it in unit testing?
Labels:
.Net,
.NET Core,
Interview Question,
Unit Test
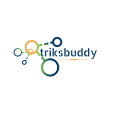
Subscribe to:
Post Comments (Atom)
No comments:
Post a Comment
Please keep your comments relevant.
Comments with external links and adult words will be filtered.